the others/Algorithm
Binary Search Tree ๊ตฌํ : pre-order / in-order / post-order
ciocio
2021. 9. 23. 23:42
๐ Binary Search Tree
class BinarySearchTree {
constructor(value){
this.value = value;
this.left = null;
this.right = null;
}
insert(value) {
if(value < this.value){
if(this.left === null){
this.left = new BinarySearchTree(value);
}
else{
this.left.insert(value);
}
}
else if(value > this.value){
if(this.right === null){
this.right = new BinarySearchTree(value);
}
else{
this.right.insert(value);
}
}
}
contains(value) {
if(value === this.value){
return true;
}
else if(this.left === null || this.right === null){
return false;
}
if(value < this.value){
return (this.left && this.left.contains(value));
}
if(value > this.value){
return (this.right && this.right.contains(value));
}
}
preorder(callback) {
callback(this.value);
if(this.left){
this.left.preorder(callback);
}
if(this.right){
this.right.preorder(callback);
}
}
inorder(callback) {
if(this.left){
this.left.inorder(callback);
}
callback(this.value);
if(this.right){
this.right.inorder(callback);
}
}
postorder(callback) {
if(this.left){
this.left.postorder(callback);
}
if(this.right){
this.right.postorder(callback);
}
callback(this.value);
}
}
let arr = [];
let callback = function (value) {
arr.push(value);
};
const rootNode = new BinarySearchTree(10);
rootNode.insert(8);
rootNode.insert(3);
rootNode.insert(7);
rootNode.insert(5);
rootNode.insert(15);
rootNode.insert(16);
rootNode.insert(11);
rootNode.insert(14);
rootNode.postorder(callback);
๐ Tree
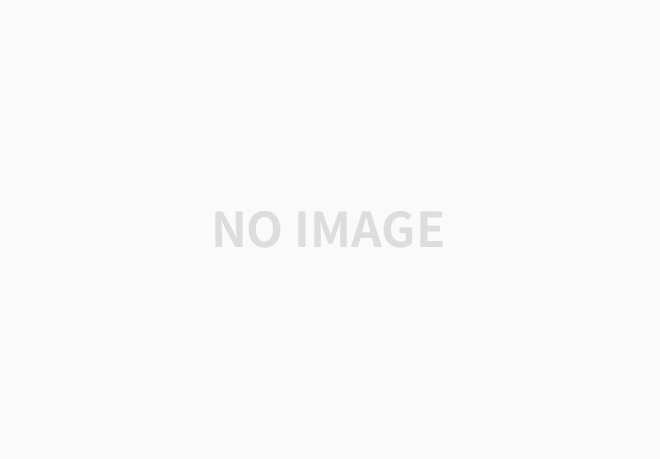
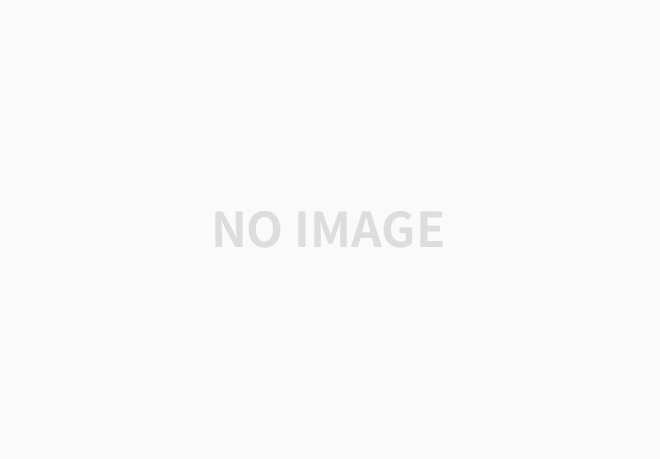
๐ Tree Traversal : preorder
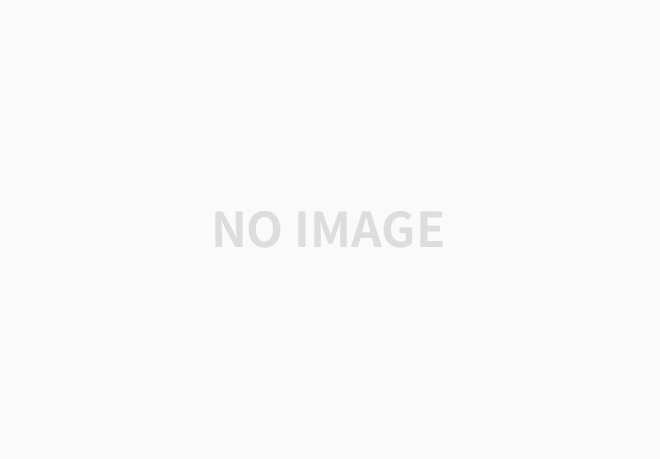
์ผ์ชฝ๋ถํฐ ๊ฒ์ฌํ๋, (๋ฃจํธ ๋ ธ๋์์ ์์ํด์) ๋ง๋๋ ๋ ธ๋ ์กฑ์กฑ ๊ฒ์ฌ ์ํ ํ ์ค๋ฅธ์ชฝ์ผ๋ก ๋์ด๊ฐ๋ ํํ
๐ Tree Traversal : inorder
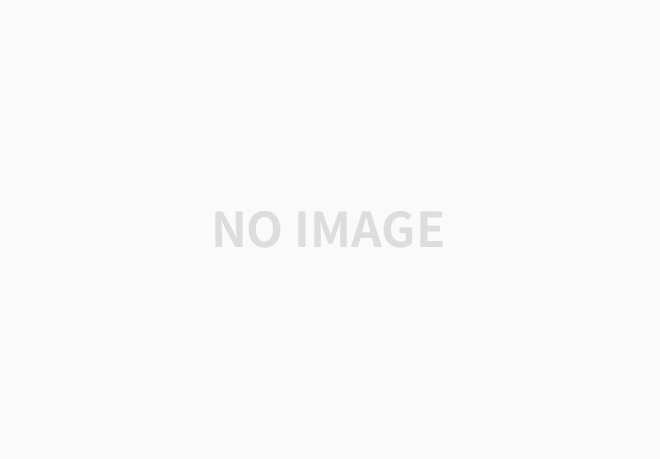
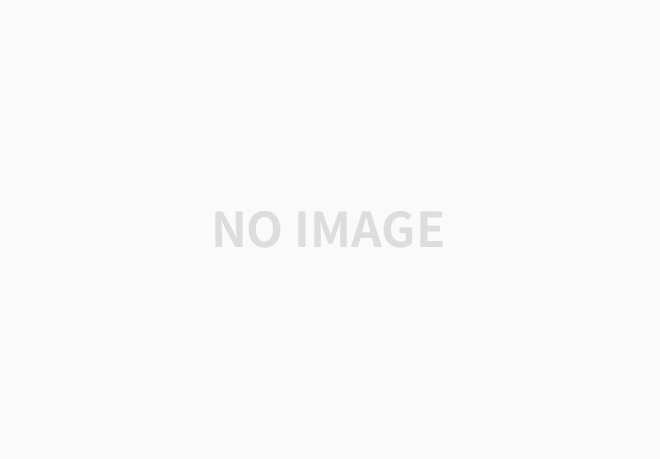
์ ์ผ ์์ ์ผ์ชฝ ๋ ธ๋๋ถํฐ ์ ์ผ ํฐ ์ค๋ฅธ์ชฝ ๋ ธ๋๊น์ง ๋์ดํ๋ ํํ
๐ Tree Traversal : postorder
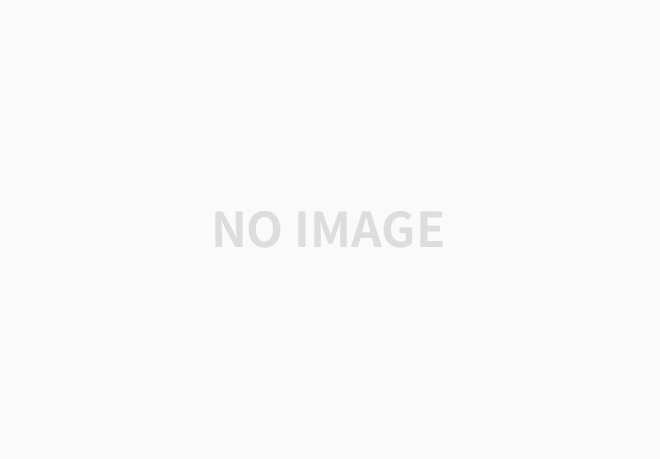
์ผ์ชฝ๋ถํฐ ๊ฒ์ฌํ๋, (๊ฐ์ฅ ๋ง๋จ ๋ ธ๋์์ ์์ํด์) ๋ง๋๋ ๋ ธ๋ ์กฑ์กฑ ๊ฒ์ฌ ์ํ ํ ์ค๋ฅธ์ชฝ์ผ๋ก ๋์ด๊ฐ๋ ํํ
preorder์ ๋ค๋ฅธ ์ ์, ๋ฃจํธ ๋ ธ๋๋ฅผ ๊ฐ์ฅ ๋ง์ง๋ง์ ๊ฒ์ฌํ๋ค๋ ์ ์ด๋ค. (์ค๊ฐ์ ๊ฑฐ์น์ง ์์)
๋ฐ์ํ